When a user clicks on a link to a file, the web browser usually decides how to handle it. Some file types, such as images, videos, or PDFs, are often displayed directly in the browser instead of being downloaded. To force the browser to download a file instead of opening it, the Content-Disposition
HTTP header can be utilized.
Understanding the Content-Disposition Header
The Content-Disposition
header tells the browser how to handle the response. It has two main values:
- inline – The file is displayed within the browser if possible.
- attachment – The file is downloaded instead of being displayed.
How to Use Content-Disposition to Force Download
To ensure a file is downloaded, the server must send the correct HTTP headers. Below is an example of how to use this header:
Content-Disposition: attachment; filename="example.pdf"
This instructs the browser to present a download option for example.pdf rather than displaying it. The filename
parameter suggests a default name for the downloaded file.
Example Implementation in Various Languages
PHP
In a PHP script, the headers can be set as follows:
header('Content-Type: application/pdf'); header('Content-Disposition: attachment; filename="example.pdf"'); readfile('example.pdf');
This script ensures that example.pdf is downloaded instead of being opened in the browser.
Apache .htaccess
Apache users can force file downloads using .htaccess
:
Header set Content-Disposition attachment
This forces the download of files with specified extensions.
Node.js with Express
In a Node.js application using Express, a similar effect can be achieved:
app.get('/download', function(req, res) { res.setHeader('Content-Disposition', 'attachment; filename="file.txt"'); res.sendFile(__dirname + '/file.txt'); });
This approach ensures that file.txt is downloaded when accessed.
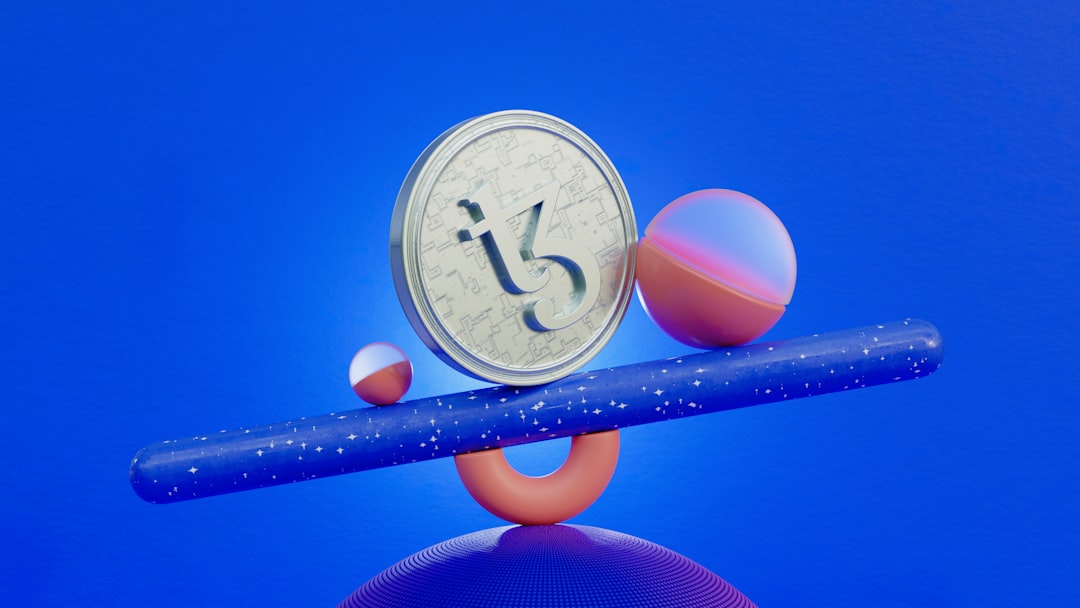
Best Practices for Using Content-Disposition
- Ensure the correct MIME type is set using
Content-Type
. - Use the
filename
attribute responsibly to suggest a reasonable file name. - Validate and sanitize file names before sending them in headers.
- Test across different browsers to ensure expected behavior.
Common Issues and Troubleshooting
Despite setting Content-Disposition: attachment
, the behavior may vary depending on browser settings. Some users may have configured their browsers to open certain file types automatically. In such cases:
- Ensure the file is not embedded within an iframe.
- Try adding the
Content-Type
explicitly. - Configure server settings to prevent overriding
Content-Disposition
.
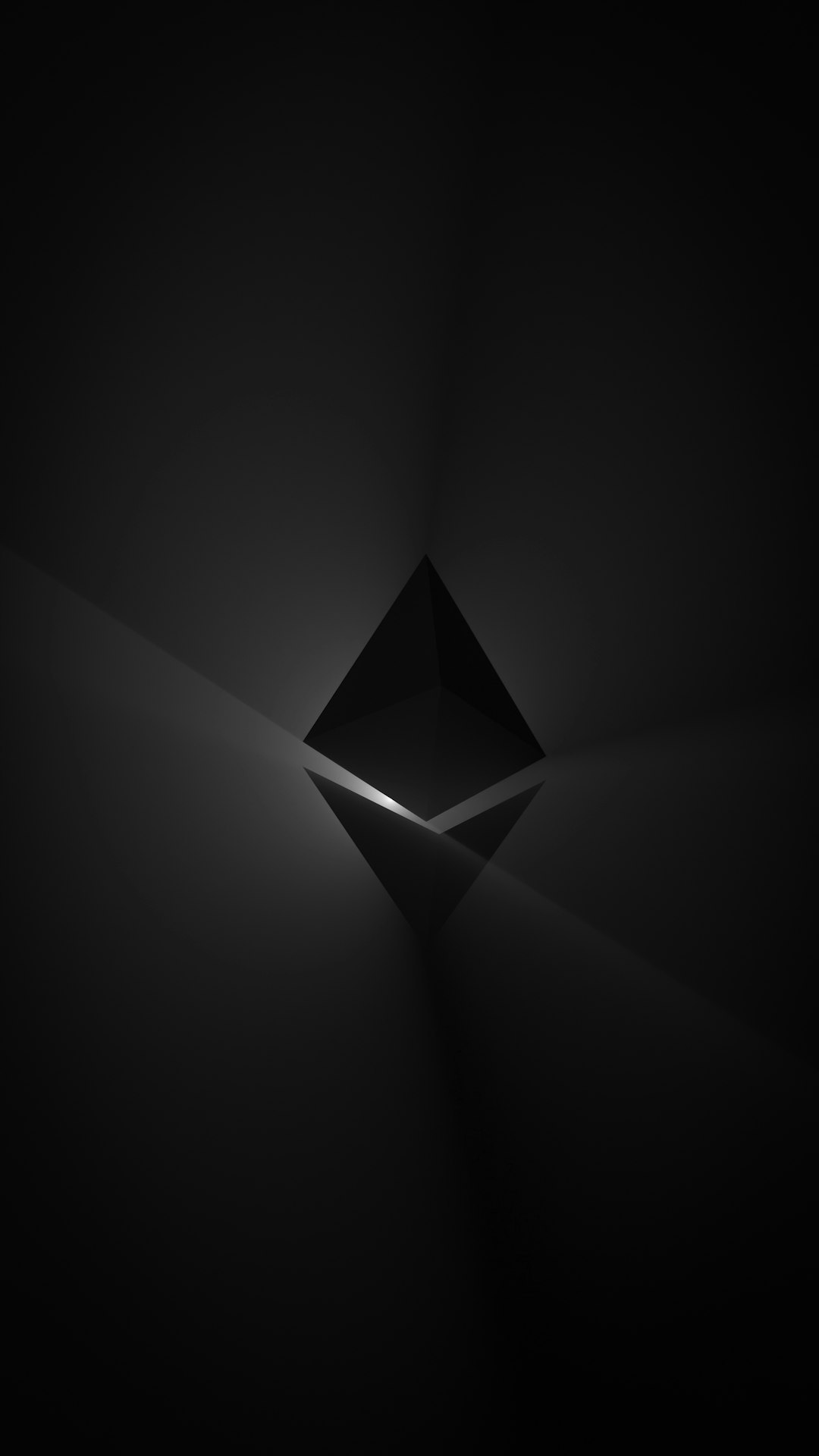
FAQ
Why do some files still open in the browser instead of downloading?
This can happen because of browser settings that automatically open certain file types based on MIME types or user preferences.
Can I force any file to be downloaded?
Yes, most types of files can be forced to download using Content-Disposition: attachment
, but browser configurations may still affect behavior.
What happens if I don’t specify a filename?
The browser may use the URL’s last portion as the default file name, which may not be user-friendly.
Does Content-Disposition work in all browsers?
Yes, but some older browsers might have inconsistent behavior. It is always recommended to test in different browsers.
Can I set Content-Disposition in JavaScript?
No, it must be set on the server side via HTTP headers.