Passing parameters into a URL in Python is super useful. Imagine you’re asking a website for information, like searching Google. You type something in the search box, and it appears in the URL. That’s exactly what we’re doing here, but with Python!
Why Pass Parameters?
Sometimes, when making a request to a website, you need to send extra information. For example:
- Searching for specific data
- Filtering results
- Accessing specific pages
This is done using query parameters in the URL.
Basic Example
Let’s use Python’s requests library to send a URL with parameters.
import requests url = "https://api.example.com/search" params = {"q": "python", "page": 2} response = requests.get(url, params=params) print(response.url) # See the final URL
This generates a URL like:
https://api.example.com/search?q=python&page=2
Nice and simple!
What’s Happening Here?
- We set the base URL.
- We create a dictionary with our parameters.
- We pass it to requests.get().
- Python adds them to the URL automatically!
Handling Special Characters
URLs don’t like special characters like spaces or “&”. Luckily, Python takes care of this.
params = {"query": "python requests", "sort": "newest"}
Python converts spaces to %20. The final URL might look like:
https://api.example.com/search?query=python%20requests&sort=newest
Adding Multiple Parameters
Adding more parameters? No problem! Just extend the dictionary.
params = { "category": "books", "author": "J.K. Rowling", "sort": "popularity" }
Your request URL now has three parameters included.
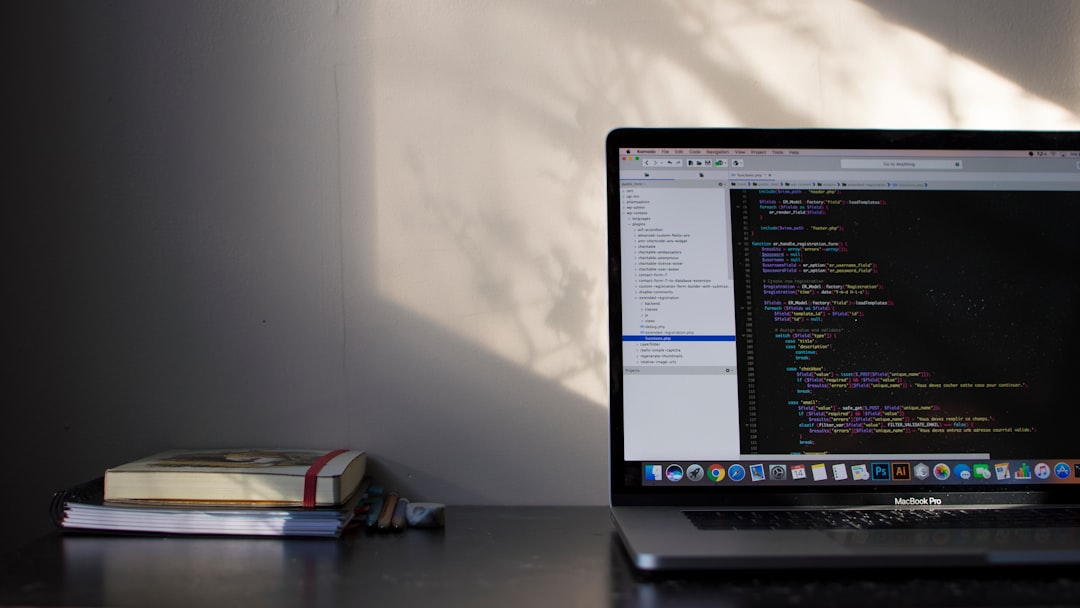
Using Parameters in POST Requests
For POST requests, parameters go in the body instead of the URL.
response = requests.post(url, data=params)
This sends data without cluttering the URL.
Handling API Keys
Some APIs require an API key for authentication. You can pass it as a parameter.
params = { "api_key": "your_secret_key", "query": "machine learning" } response = requests.get(url, params=params)
Make sure to keep your API keys private!
Error Handling
What if something goes wrong? Always check the response.
if response.status_code == 200: print("Success!") else: print("Error:", response.status_code)
This prevents unnecessary headaches.
Encoding Parameters Manually
Sometimes, you need more control over encoding.
import urllib.parse query = {"title": "Harry Potter & the Philosopher's Stone"} encoded_query = urllib.parse.urlencode(query) print(encoded_query) # Output: title=Harry+Potter+%26+the+Philosopher%27s+Stone
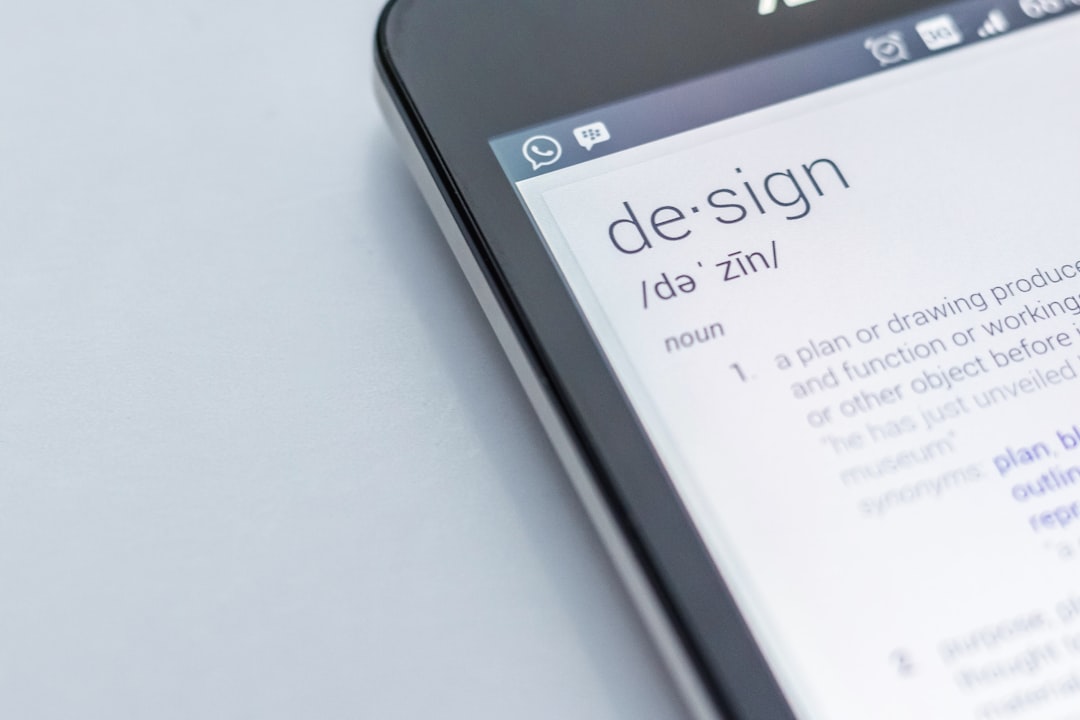
Final Thoughts
Passing parameters in URLs is easy with Python’s requests module. Just remember:
- Use dictionaries to pass parameters.
- Python automatically encodes special characters.
- Check for errors in responses.
Now go ahead and experiment with your own requests!